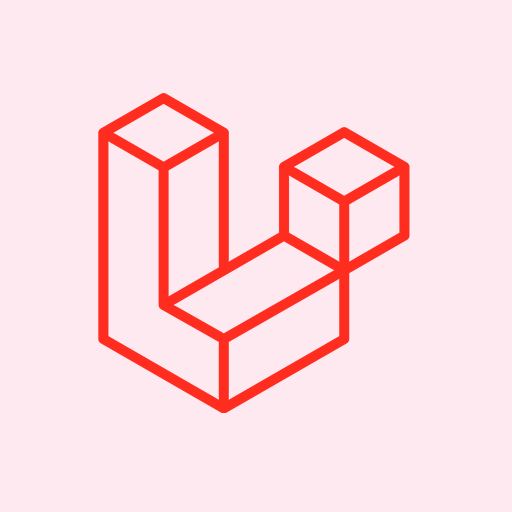
Laravel
Laravel is a web application framework with expressive, elegant syntax.
Laravel Cheat Sheet for Eloquent ORM
ORM (Object-relational mapping) is used to make database CRUD operations easier. Laravel comes with Eloquent ORM. This tutorial is created to provide some of the frequently used cheat sheet for Larave
- 6 years ago
- 12.3K Views
Scheduling Tasks with Cron Job in Laravel 5.8
Cron Job is used to schedule tasks that will be executed every so often. Crontab is a file that contains a list of scripts, By editing the Crontab, You can run the scripts periodically.
- 6 years ago
- 8.6K Views