Posts
Laravel
PHP
Linux
Python
Ubuntu
Android
React Native
Digital Ocean
JavaScript
Programming
WordPress
AWS
Apple
Vue.js
Windows
Tech
Book Review
Breaking Down Monoliths with Module Federation: A Vue + Vite Deep Dive
Is your frontend application starting to feel like a monolithic beast? Are development cycles slowing down, and teams stepping on each other's toes? If you answered yes, Module Federation is there
- 2 months ago
- 471 Views
My take on How China is Revolutionizing Technology
China is at the forefront of a technological revolution, reshaping industries globally through groundbreaking innovation and rapid adoption. From artificial intelligence to renewable energy.
- 3 months ago
- 680 Views
GraphQL in Laravel Using Lighthouse
In modern web development, GraphQL has emerged as a powerful alternative to REST APIs due to its flexibility and efficiency.
- 10 months ago
- 1.7K Views
Sanitize and Create URLs via Laravel command
Clean, legible URLs are essential for modern web applications, as they improve user experience and search engine optimisation. Using custom Artisan commands in Laravel 11.
- 11 months ago
- 1.8K Views
Short Summary : The Obstacle is the way
The Obstacle is the way by Ryan Holiday is based on the school of philosophy known as Stoicism which helps to lay the foundation of overcoming the obstacles and turning them into opportunity.
- 3 years ago
- 3K Views
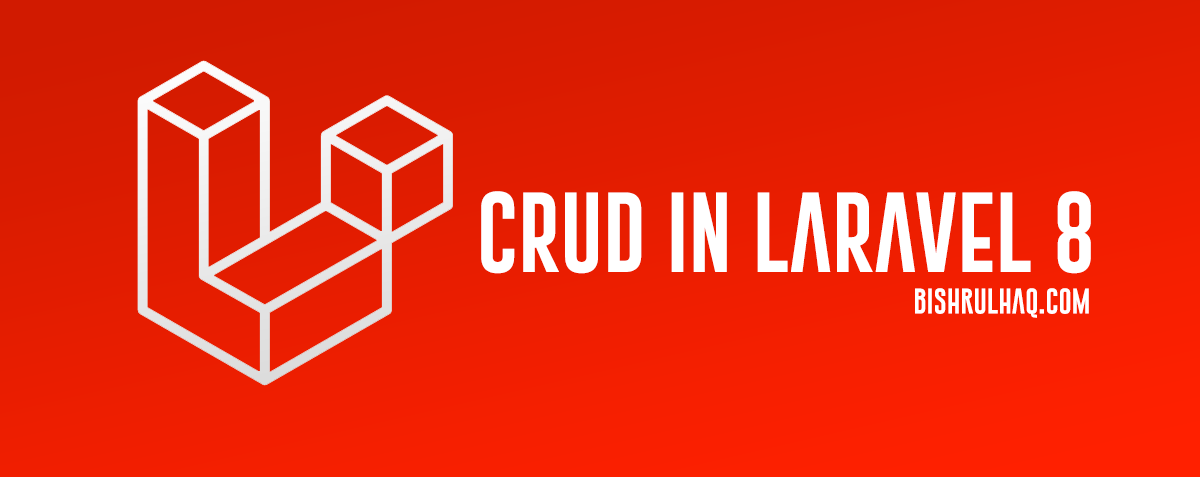
CRUD Operations In Laravel 8
This tutorial is created to illustrate the basic CRUD (Create , Read, Update, Delete) operation using SQL with Laravel 8. Laravel is one of the fastest-growing frameworks for PHP.
- 4 years ago
- 6.7K Views
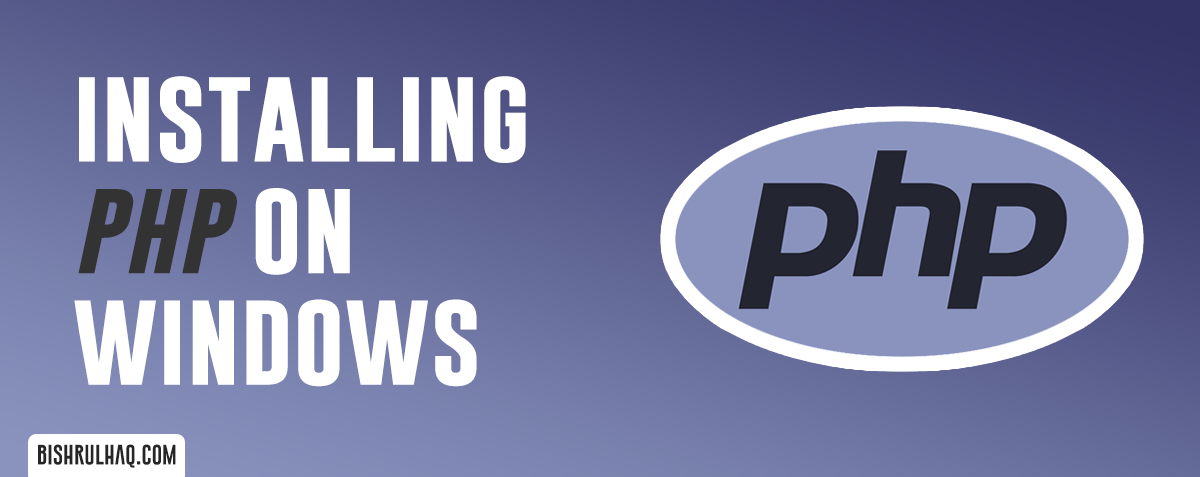
Installing PHP on Windows
PHP is known to be one of the most commonly used server-side programming language on the web. It provides an easy to master with a simple learning curve. It has close ties with the MySQL database.
- 4 years ago
- 2.8K Views
Important functionalities of Pandas in Python : Tricks and Features
Pandas is one of my favorite libraries in python. It’s very useful to visualize the data in a clean structural manner. Nowadays Pandas is widely used in Data Science, Machine Learning and other areas.
- 5 years ago
- 6.4K Views